---- ISSUE SOLVED ---
I work with our devops team again, and the solution for this issue was to add the domain certificate to the machine trusted certificates
--- END UPDATE ---
Hi,
I am writing a C# wiform app that should upload files to MinIO.
We have 2 MinIO storages:
- One stored on Azure and accessed via external IP
- The second stored in our internal VM which i access it via internal IP
I face issues working with the MinIO storage stored on our internal VM.
It looks like the MinIO storages are the same (I went through the settings on the MinIO web interface).
I worked with our DevOps expert, and he does not see any issues or different configurations done in the storages.
The addresses are correct. The access key and secret key are correct.
Accessing the MinIO storage via our node microservices, which run on Kubernetes, is working with no issue.
Accessing the MinIO storage from our c# web application, which runs on Windows Server IIS, is working with no issue.
Accessing the MinIO storage using our c# web application, which run on Windows machine IIS, and run on the same machine i run this test app, is working. If i run the code on the web app unit testing, it failes as well.
Running the C# WinForm application from the same machine I run the web app on is failing.
I tried to use both MinIO V4 and V6 from nugets.
I tried to upload a file (using PutObjectAsync) and list the buckets in the storage (using ListBucketsAsync).
On MinIO V4, I got the following error (AggregateException):
MinIO API responded with message=Connection error: An error occurred while sending the request. Status code=0, response=An error occurred while sending the request., content=
On MinIO V6, I got no error, but nothing happened (I failed to upload a file or get the bucket list).
I built a function that opens a connection to port 443 to see if the IP and port are open, and it is working as expected.
I run my test application as administrator
I been able to load file via the Web Interface of the MinIO storage
I wrote a simple app (attached bellow).
screen shot of the MinIO web interface attached
appriciate your help here
Nachum
The Working MinIO Web Interface
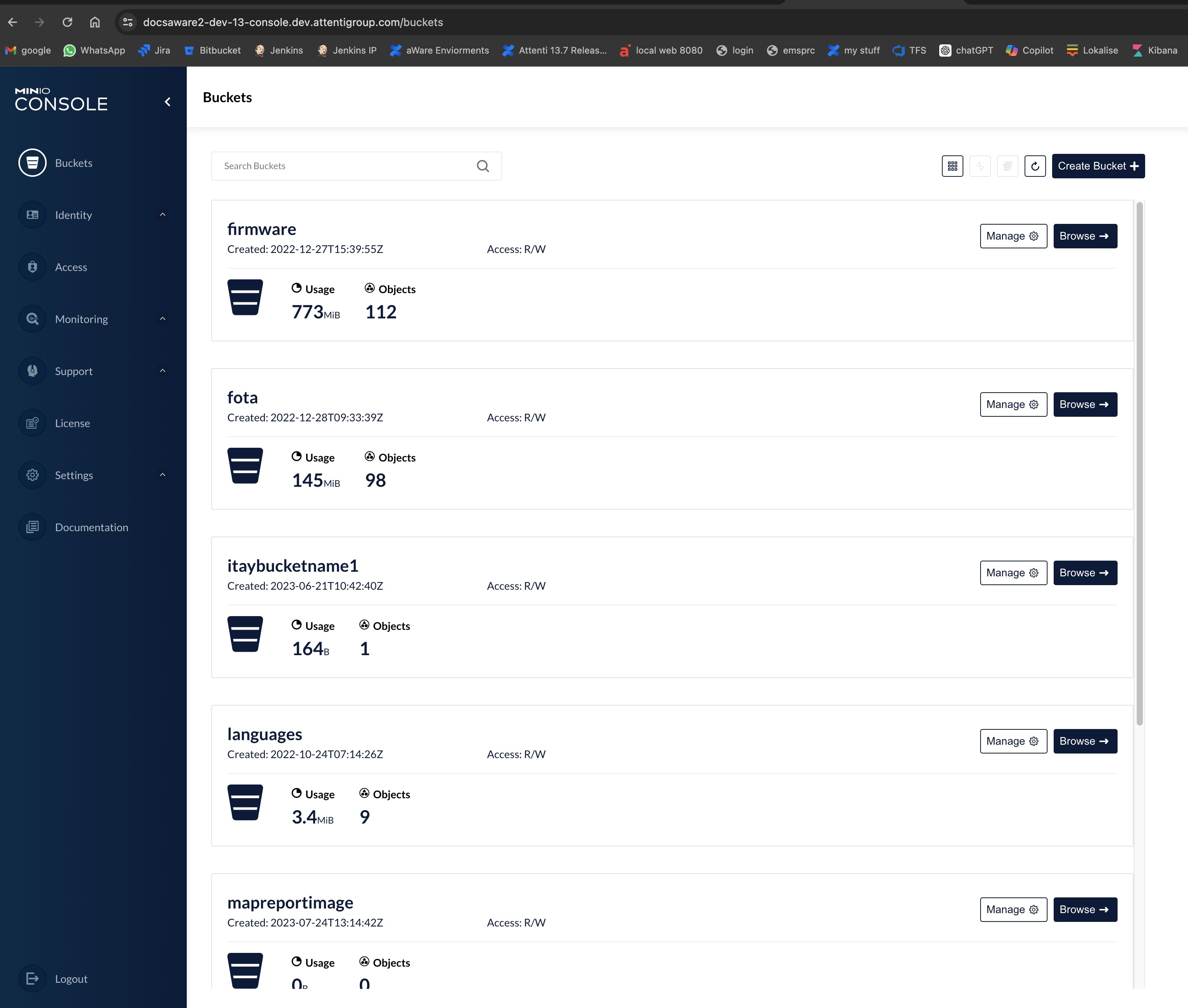
The not working MinIO web interface
Not working site.jpeg
The code i use
using Minio;
using Minio.DataModel;
using Minio.Exceptions;
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Net.Sockets;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace test
{
public partial class Form1 : Form
{
List<string> siteslist = new List<string>()
{
"docsrnd2.attentigroup.local",
"docsaware2-dev-13.dev.attentigroup.com"
};
public Form1()
{
InitializeComponent();
comboBoxEndpoints.Items.Clear();
comboBoxEndpoints.DataSource = siteslist;
}
private void CheckConnection(string ip, int port)
{
TcpClient tcpClient = new TcpClient();
var res = tcpClient.BeginConnect(ip, port, null, null);
var success = res.AsyncWaitHandle.WaitOne(TimeSpan.FromSeconds(2));
if (success)
{
Socket sock = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
sock.Connect(ip, port);
if (sock.Connected == true) // Port is in use and connection is successful
{
//result = "";
}
sock.Close();
}
else
{
throw new Exception($"Connection to {ip}:{port} failed.");
}
tcpClient.Close();
}
private void UploadFileToMinio()
{
string endpoint = comboBoxEndpoints.Text;
string accessKey = "accesskey";
string secretKey = "secretkey";
var bucketName = "languages";
var objectName = "en-GB-test.json";
var filePath = "en_GB.json";
var contentType = "application/zip";
try
{
CheckConnection(endpoint, 443);
MinioClient minio = new MinioClient()
.WithEndpoint(endpoint)
.WithCredentials(accessKey, secretKey)
.WithSSL()
.Build();
Task task;
try
{
// Upload a file to bucket.
var putObjectArgs = new PutObjectArgs()
.WithBucket(bucketName)
.WithObject(objectName)
.WithFileName(filePath)
.WithContentType(contentType);
task = minio.PutObjectAsync(putObjectArgs);
task.Wait();
MessageBox.Show($"file successfully uploaded to {endpoint} accessKey: {accessKey}, secretKey: {secretKey}, {bucketName}/{objectName}");
}
catch (MinioException mex)
{
MessageBox.Show($"file fail uploaded to {endpoint} accessKey: {accessKey}, secretKey: {secretKey}, {bucketName}/{objectName}. Error: {mex.Message}");
}
catch (Exception ex)
{
MessageBox.Show($"file fail uploaded to {endpoint} accessKey: {accessKey}, secretKey: {secretKey}, {bucketName}/{objectName}. Error: {ex.Message}");
}
}
catch (Exception ex)
{
MessageBox.Show($"file fail uploaded to {endpoint} accessKey: {accessKey}, secretKey: {secretKey}, {bucketName}/{objectName}. Error: {ex.Message}");
}
}
public void GetBucketsList()
{
string endpoint = comboBoxEndpoints.Text;
string accessKey = Properties.Settings.Default.accessKey;
string secretKey = Properties.Settings.Default.secretKey;
try
{
CheckConnection(endpoint, 443);
MinioClient minio = new MinioClient()
.WithEndpoint($"{endpoint}")
.WithCredentials(accessKey, secretKey)
.WithSSL()
.Build();
var getListBucketsTask = minio.ListBucketsAsync();
Task.WaitAll(getListBucketsTask); // block while the task completes
var list = getListBucketsTask.Result;
if (list.Buckets?.Count > 0)
{
var buckets = list.Buckets;
string result = "\n";
foreach (var bucket in list.Buckets)
{
result += bucket.Name + " " + bucket.CreationDateDateTime + "\n";
}
MessageBox.Show($"buckets list from {endpoint} accessKey: {accessKey}, secretKey: {secretKey}{result}");
}
else
{
MessageBox.Show($"buckets list is empty {endpoint} accessKey: {accessKey}, secretKey: {secretKey}");
}
}
catch (AggregateException aggEx)
{
MessageBox.Show($"fail get buckets list from {endpoint} accessKey: {accessKey}, secretKey: {secretKey}. error: {aggEx.Message}, {aggEx.InnerException.Message} ");
}
catch (InvalidEndpointException iepEx)
{
MessageBox.Show($"fail get buckets list from {endpoint} accessKey: {accessKey}, secretKey: {secretKey}. error: {iepEx.Message}");
}
catch (Exception ex)
{
MessageBox.Show($"fail get buckets list from {endpoint} accessKey: {accessKey}, secretKey: {secretKey}. error: {ex.Message}");
}
}
private void button1_Click(object sender, EventArgs e)
{
UploadFileToMinio();
}
private void button2_Click(object sender, EventArgs e)
{
GetBucketsList();
}
private void button3_Click(object sender, EventArgs e)
{
Application.Exit();
}
}
}