you will need a postback page in site hosting the Blazor WASM. it should render the Blazor host page, with the postback data serialized to a global javascript variable. on startup the Blazor app should use jsinterop to check for this data. if found it should also restore the saved state before redirecting to the payment application.
How to insert HTML to a Blazor WASM?
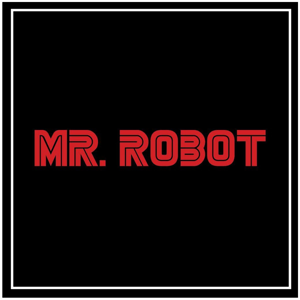
I am working on an e-commerce solution. The client side is a Blazor WASM .Net 6 project. I am trying to use a 3rd party payment gateway and unfortunately, their support is very poor. I am trying to progress by trial and error. On the checkout razor page, I am trying to inject a script that displays credit card info on the page which I am getting this script as a response from the 3rd party gateway API. Here is the sample script content and the display (https://codebeautify.org/htmlviewer#):
@page "/ECommerce/Checkout"
@page "/ECommerce/Checkout/{StandardDeliveryCharge:double}"
@inject IJSRuntime _jsRuntime
@inject ILocalStorageService _localStorage
@inject IProductService _productService
@inject IPaymentService _paymentService
@inject IOrderService _orderService
@inject NavigationManager _navigationManager
@using Microsoft.AspNetCore.Authorization
@attribute [Authorize]
@if (IsProcessing)
{
<div style="position:fixed;top:50%;left:50%;margin-top:-50px;margin-left:-100px;">
<img src="images/Spinner@1x-1.0s-200px-200px.gif" alt="waiting" />
</div>
}
else
{
<div id="iyzipay-checkout-form" class="responsive"></div>
<div class="untree_co-section">
<div class="container">
<EditForm Model="Order.OrderHeader" OnValidSubmit="HandleCheckout">
<DataAnnotationsValidator/>
<div class="row">
<div class="col-md-6 mb-5 mb-md-0">
<h2 class="h3 mb-3 text-black">Billing Details</h2>
<div class="p-3 p-lg-5 border bg-white">
<div class="form-group row">
<div class="col-md-12">
<label for="c_fname" class="text-black">First Name <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.Name" type="text" class="form-control" id="c_fname" name="c_fname"/>
<ValidationMessage For="() => Order.OrderHeader.Name"></ValidationMessage>
</div>
</div>
<div class="form-group row">
<div class="col-md-12">
<label for="c_address" class="text-black">Address <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.StreetAddress" type="text" class="form-control" id="c_address" name="c_address" placeholder="Street address"/>
<ValidationMessage For="() => Order.OrderHeader.StreetAddress"></ValidationMessage>
</div>
</div>
<div class="form-group mt-3">
<label for="c_city" class="text-black">City <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.City" type="text" class="form-control" id="c_city" name="c_city" placeholder="City"/>
<ValidationMessage For="() => Order.OrderHeader.City"></ValidationMessage>
</div>
<div class="form-group row">
<div class="col-md-6">
<label for="c_state_country" class="text-black">State / Country <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.State" type="text" class="form-control" id="c_state_country" name="c_state_country"/>
<ValidationMessage For="() => Order.OrderHeader.State"></ValidationMessage>
</div>
<div class="col-md-6">
<label for="c_postal_zip" class="text-black">Posta / Zip <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.PostalCode" type="text" class="form-control" id="c_postal_zip" name="c_postal_zip"/>
<ValidationMessage For="() => Order.OrderHeader.PostalCode"></ValidationMessage>
</div>
</div>
<div class="form-group row mb-5">
<div class="col-md-6">
<label for="c_email_address" class="text-black">Email Address <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.Email" type="text" class="form-control" id="c_email_address" name="c_email_address"/>
<ValidationMessage For="() => Order.OrderHeader.Email"></ValidationMessage>
</div>
<div class="col-md-6">
<label for="c_phone" class="text-black">Phone <span class="text-danger">*</span></label>
<InputText @bind-Value="Order.OrderHeader.PhoneNumber" type="text" class="form-control" id="c_phone" name="c_phone" placeholder="Phone Number"/>
<ValidationMessage For="() => Order.OrderHeader.PhoneNumber"></ValidationMessage>
</div>
</div>
</div>
</div>
<div class="col-md-6">
<div class="row mb-5">
<div class="col-md-12">
<h2 class="h3 mb-3 text-black">Your Order</h2>
<div class="p-3 p-lg-5 border bg-white">
<table class="table site-block-order-table mb-5">
<thead>
<th>Product</th>
<th>Total</th>
</thead>
<tbody>
@foreach (var prod in Order.OrderDetails)
{
<tr>
<td>
@prod.ProductName <strong class="mx-2">x</strong> @prod.Count
</td>
<td>@prod.Price.ToString("c")</td>
</tr>
}
<tr>
<td class="text-black font-weight-bold">
<strong>Cart Subtotal (Shipment)</strong>
</td>
<td class="text-black">@StandardDeliveryCharge.ToString("c")</td>
</tr>
<tr>
<td class="text-black font-weight-bold">
<strong>Order Total</strong>
</td>
<td class="text-black font-weight-bold">
<strong>@((Order.OrderHeader.OrderTotal + @StandardDeliveryCharge).ToString("c"))</strong>
</td>
</tr>
</tbody>
</table>
<label for="dropdownMenuButton1" class="payment">Payment Method</label>
<div class="col-md-12">
<div class="btn-group dropend mt-2">
<button type="button" class="btn btn-secondary dropdown-toggle" data-bs-toggle="dropdown" aria-expanded="false" id="dropdownMenuButton1">
@SelectedPayment
</button>
<ul class="dropdown-menu dropdown-menu-dark">
<li>
<h5 class="dropdown-header">Available Payment Methods</h5>
</li>
<li>
<a class="dropdown-item" @onclick="@(() => SelectPaymentMethod("Stripe"))">Stripe Payment</a>
</li>
<li>
<hr class="dropdown-divider">
</li>
<li>
<a class="dropdown-item" @onclick="@(() => SelectPaymentMethod("Iyzico"))">Iyzipay Payment</a>
</li>
</ul>
</div>
<div class="form-group mt-5">
<button class="btn btn-black btn-lg py-3 btn-block" type="submit">Place Order</button>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</EditForm>
<!-- </form> -->
</div>
</div>
}
@code {
private string checkout;
private string SelectedPayment { get; set; } = "Stripe";
private SuccessModelDTO result = new SuccessModelDTO()
{
StatusCode = 0
};
private async Task HandleCheckout()
{
if(SelectedPayment == "Stripe")
{
await StripePayment();
}
else if (SelectedPayment == "Iyzico")
{
await IyzicoPayment(Order);
}
}
private async Task IyzicoPayment(OrderDTO order)
{
try
{
IsProcessing = true;
var iyzicoPaymentDto = new IyzicoPaymentDTO()
{
Order = Order
};
result = await _paymentService.Checkout(iyzicoPaymentDto);
if(result.StatusCode == 200)
{
checkout = result.Data.ToString();
var orderDtoSaved = await _orderService.CreateForIyzico(iyzicoPaymentDto);
await _localStorage.SetItemAsync(SD.Local_OrderDetails, orderDtoSaved);
await _jsRuntime.InvokeVoidAsync("injectIyzicoScript", checkout);
}
IsProcessing = false;
}
catch (Exception e)
{
await _jsRuntime.ToastrError(e.Message);
}
}
}
Here is the javascript that I am trying to inject script:
function injectIyzicoScript(scriptContent) {
const div = document.getElementById("iyzipay-checkout-form");
if (div != null) {
div.insertAdjacentHTML("beforeend", scriptContent);
} else {
console.error('Element with id "iyzipay-checkout-form" not found.');
}
}
But I am getting Element with id "iyzipay-checkout-form" not found.
on the browser console and there is no card info page displayed.
Here is the sample script content coming from 3rd party API that I am trying to inject into the HTML.
<script type="text/javascript">if (typeof iyziInit == 'undefined') {var iyziInit = {currency:"TRY",token:"dce6b28f-f7c6-45b0-af50-30473899f11f",price:1000.00,pwiPrice:1000.00,locale:"tr",baseUrl:"https://sandbox-api.iyzipay.com", merchantGatewayBaseUrl:"https://sandbox-merchantgw.iyzipay.com", registerCardEnabled:true,bkmEnabled:true,bankTransferEnabled:false,bankTransferTimeLimit:{"value":5,"type":"day"},bankTransferRedirectUrl:"http://localhost:7169/OrderConfirmation",bankTransferCustomUIProps:{},campaignEnabled:false,campaignMarketingUiDisplay:null,paymentSourceName:"",plusInstallmentResponseList:null,payWithIyzicoSingleTab:false,payWithIyzicoSingleTabV2:false,payWithIyzicoOneTab:false,newDesignEnabled:false,mixPaymentEnabled:true,creditCardEnabled:true,bankTransferAccounts:[],userCards:[],fundEnabled:true,memberCheckoutOtpData:{},force3Ds:false,isSandbox:true,storeNewCardEnabled:true,paymentWithNewCardEnabled:true,enabledApmTypes:["SOFORT","IDEAL","QIWI","GIROPAY"],payWithIyzicoUsed:false,payWithIyzicoEnabled:true,payWithIyzicoCustomUI:{},buyerName:"John",buyerSurname:"Doe",merchantInfo:"",merchantName:"Sandbox Merchant Name - 3397951",cancelUrl:"",buyerProtectionEnabled:false,hide3DS:false,gsmNumber:"",email:"email@email.com",checkConsumerDetail:{},subscriptionPaymentEnabled:false,ucsEnabled:false,fingerprintEnabled:false,payWithIyzicoFirstTab:false,creditEnabled:false,payWithIyzicoLead:false,goBackUrl:"",metadata : {},createTag:function(){var iyziJSTag = document.createElement('script');iyziJSTag.setAttribute('src','https://sandbox-static.iyzipay.com/checkoutform/v2/bundle.js?v=1719940949305');document.head.appendChild(iyziJSTag);}};iyziInit.createTag();}</script>
How can I do this?
3 answers
Sort by: Most helpful
-
-
Deleted
This answer has been deleted due to a violation of our Code of Conduct. The answer was manually reported or identified through automated detection before action was taken. Please refer to our Code of Conduct for more information.
Comments have been turned off. Learn more
-
Deleted
This answer has been deleted due to a violation of our Code of Conduct. The answer was manually reported or identified through automated detection before action was taken. Please refer to our Code of Conduct for more information.
Comments have been turned off. Learn more