Developing A Custom VMConnect Application
Some folks have asked creating there own VMConnect application – it’s actually really easy. Here’s a very basic example of how to create your own VMConnect application. You would likely want to add some error handling, change the window sizing, maybe a WMI call to retrieve the name of the VM vs the ID but with Hyper-V’s WMI API you can do all of that and more after all now it’s your VMConnect application.
Steps To Create Basic Application
- Create a new C# Windows Forms project In Visual Studio
- Add a COM reference to mstscax.dll (Microsoft Terminal Service Active Client 1.0 Type Library)
- Add a new RDP Client Control to the form
- From the form designer right click in the toolbox and select Choose Toolbox Item
- Select COM Components and find and check “Microsoft RDP Client Control – version 9
- From All Windows Forms (generally at the bottom) find the “Microsoft RDP Client Control – version 9” option and drag it to the form
- Add a text box for the VM ID and a connect button to the form
- For the connect button’s click event specify the following basic code
private void ConnectButton_Click(object sender, EventArgs e)
{
//specify the server the VM is running on
axMsRdpClient8NotSafeForScripting1.Server = "localhost";
//enable relative mouse mode and smart sizing
axMsRdpClient8NotSafeForScripting1.AdvancedSettings7.RelativeMouseMode = true;
axMsRdpClient8NotSafeForScripting1.AdvancedSettings7.SmartSizing = true;
//specify the authentication service - this is required and set the authentication level
axMsRdpClient8NotSafeForScripting1.AdvancedSettings7.AuthenticationServiceClass =
"Microsoft Virtual Console Service";
axMsRdpClient8NotSafeForScripting1.AdvancedSettings6.AuthenticationLevel = 0;
//retrieve the activeX control and enable CredSSP and disable NegotiateSecurity
MSTSCLib.IMsRdpClientNonScriptable3 Ocx =
(MSTSCLib.IMsRdpClientNonScriptable3)axMsRdpClient8NotSafeForScripting1.GetOcx();
Ocx.EnableCredSspSupport = true;
Ocx.NegotiateSecurityLayer = false;
//retrieve the activeX control and disable CredentialsDelegation
MSTSCLib.IMsRdpExtendedSettings rdpExtendedSettings =
(MSTSCLib.IMsRdpExtendedSettings)axMsRdpClient8NotSafeForScripting1.GetOcx();
object True = true;
rdpExtendedSettings.set_Property("DisableCredentialsDelegation", ref True);
//set the RDPPort and set the PCB string to the VM's ID
axMsRdpClient8NotSafeForScripting1.AdvancedSettings2.RDPPort = 2179;
axMsRdpClient8NotSafeForScripting1.AdvancedSettings7.PCB = vmIDTextBox.Text;
//connect to the VM
axMsRdpClient8NotSafeForScripting1.Connect();
}
Step 1 - Create a new C# Windows Forms project In Visual Studio
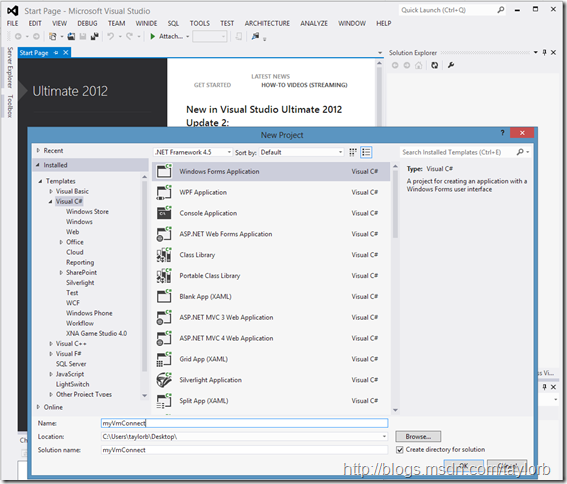
Step 2 - Add a COM reference to mstscax.dll (Microsoft Terminal Service Active Client 1.0 Type Library)
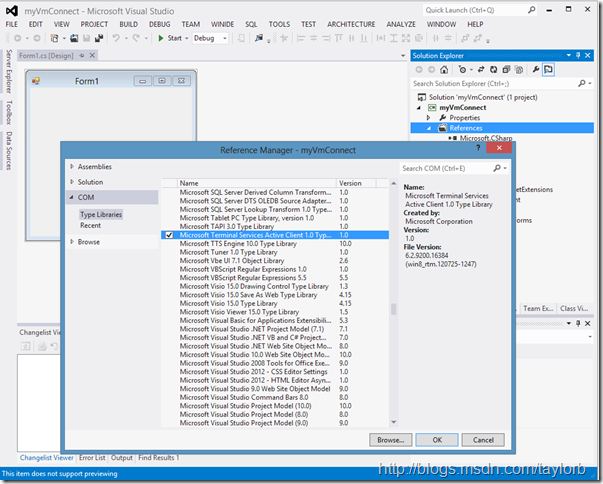
Step 3.1 - Add a new RDP Client Control to the form, From the form designer right click in the toolbox and select Choose Toolbox Item
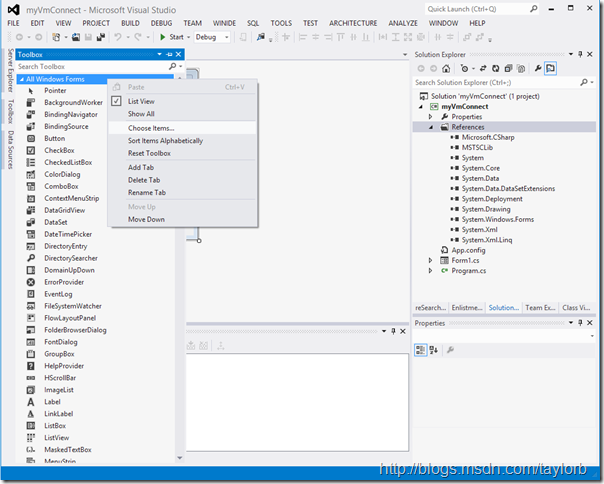
Step 3.2 - Add a new RDP Client Control to the form, Select COM Components and find and check “Microsoft RDP Client Control – version 9
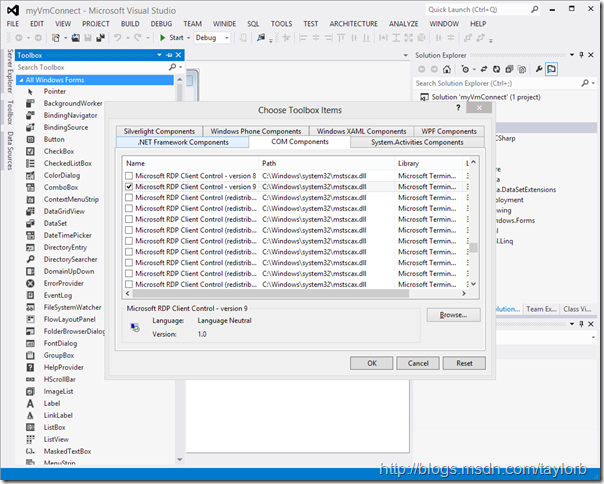
Step 3.2 - Add a new RDP Client Control to the form, From All Windows Forms (generally at the bottom) find the “Microsoft RDP Client Control – version 9” option and drag it to the form
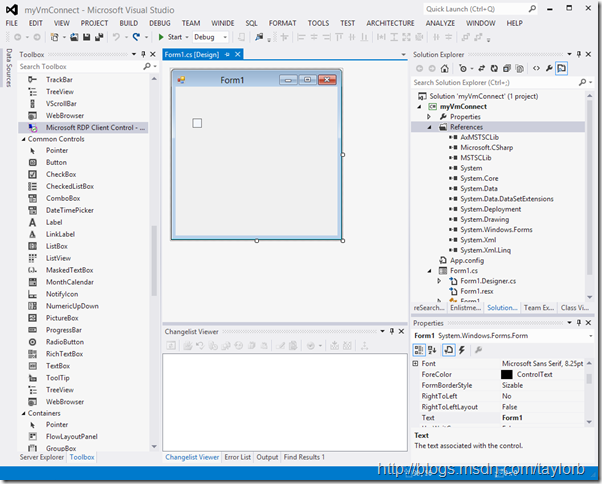
Step 4 - Add a text box for the VM ID and a connect button to the form
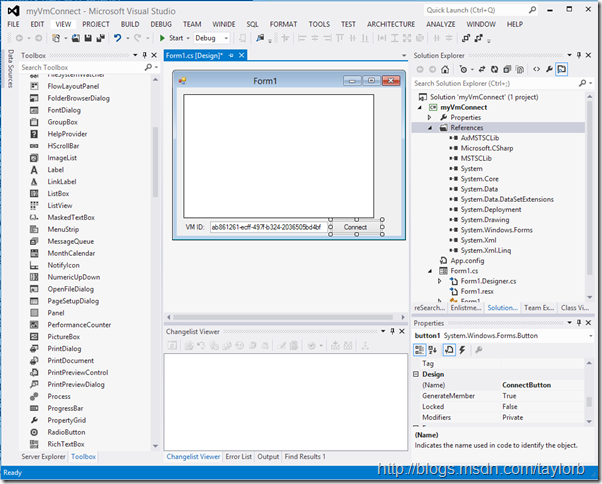
Step 5 - For the connect button’s click event specify the following basic code
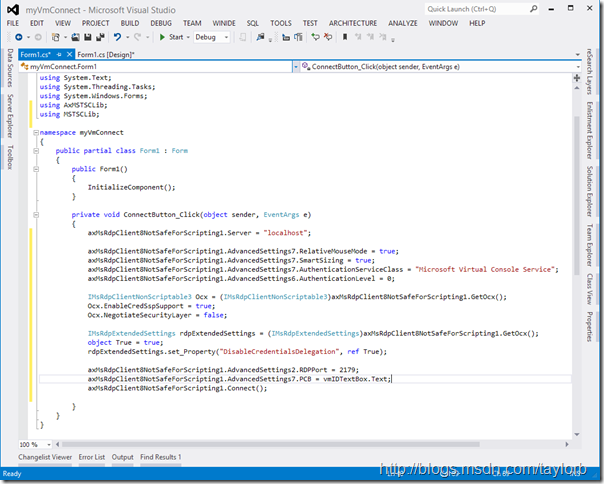
Your new solution in action!
-taylorb
Program Manager, Hyper-V
Comments
Anonymous
June 06, 2013
The comment has been removedAnonymous
June 07, 2013
Odin, I was able to reproduce the same symptoms you reported and it took me a few minutes to figure out the problem… VMConnect automatically run’s elevated as administrator. Where the custom application doesn’t have the property set (by default). Try running the app as administrator. -taylorb Program Manager, Hyper-VAnonymous
June 17, 2013
What is required to get the compiled program to run on Free Core Server 2012?? I just returns RDP.exe has stopped working and when I click on problem details I get this Problem signature: Problem Event Name: CLR20r3 Problem Signature 01: rdp.exe Problem Signature 02: 1.0.0.0 Problem Signature 03: 51a7c14d Problem Signature 04: System.Windows.Forms Problem Signature 05: 4.0.30319.17929 Problem Signature 06: 4ffa5c0e Problem Signature 07: c2b Problem Signature 08: 36 Problem Signature 09: PSZQOADHX1U5ZAHBHOHGHLDGIY4QIXHX OS Version: 6.2.9200.2.0.0.272.42 Locale ID: 1033 Additional Information 1: 5861 Additional Information 2: 5861822e1919d7c014bbb064c64908b2 Additional Information 3: dac6 Additional Information 4: dac6c2650fa14dd558bd9f448e23afd1 Read our privacy statement online: go.microsoft.com/fwlink If the online privacy statement is not available, please read our privacy statement offline: C:Windowssystem32en-USerofflps.txt However it works on 2012 GUI server?Anonymous
June 20, 2013
Never mind I found a this vmconnect.codeplex.com it uses freerdp's wfreerdp.exe and a powershell script to allow you yo replace both vmconnect.exe and mstsc,exe.Anonymous
July 22, 2013
The comment has been removedAnonymous
July 29, 2013
Thanks! Very helpful!Anonymous
October 22, 2013
Any pointers on how to configure the connection to work with the new Enhanced Session Mode in Hyper-V 2012 R2? Would love to be able to use clipboard and audio redirection!Anonymous
December 02, 2013
What other properties are available and not documented?Anonymous
March 09, 2014
How can you send CTRL+ALT+DEL to unlock this workstation? Thanks