HidDevice クラス
定義
重要
一部の情報は、リリース前に大きく変更される可能性があるプレリリースされた製品に関するものです。 Microsoft は、ここに記載されている情報について、明示または黙示を問わず、一切保証しません。
最上位のコレクションと対応するデバイスを表します。
public ref class HidDevice sealed : IClosable
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.Agile)]
class HidDevice final : IClosable
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.Agile)]
public sealed class HidDevice : System.IDisposable
Public NotInheritable Class HidDevice
Implements IDisposable
- 継承
- 属性
- 実装
Windows の要件
デバイス ファミリ |
Windows 10 (10.0.10240.0 - for Xbox, see UWP features that aren't yet supported on Xbox で導入)
|
API contract |
Windows.Foundation.UniversalApiContract (v1.0 で導入)
|
例
このクラスの使用方法を示す完全なサンプルについては、「 カスタム HID デバイスのサンプル」を参照してください。
次の例では、XAML と C# で構築された UWP アプリで GetDeviceSelector メソッドを使用して、特定の HID デバイス (この場合は Microsoft Input Configuration Device) のセレクターを作成し、 FromIdAsync メソッドを使用してそのデバイスへの接続を開く方法を示します。
Note
このスニペットは、システムに存在しない可能性がある HID デバイスの検索を試みます。 システムでコードを正常にテストするには、vendorId、productId、usagePage、usageId を有効な値で更新する必要があります。
- デバイス マネージャーを開く
- ヒューマン インターフェイス デバイスの拡張
- HID デバイスを見つけます (この例では、 Microsoft Input Configuration Device を選択しました)
- デバイスを右クリックし、[プロパティ] を選択します
- [プロパティ] で、[ 詳細 ] タブを選択します
- [詳細] タブの [プロパティ] ドロップダウンから [ハードウェア ID] を選択します。
- HID の詳細が [ 値 ] ボックスに表示されます。
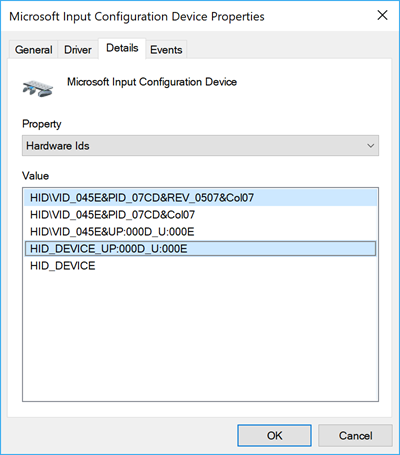
using System;
using System.Linq;
using Windows.Devices.Enumeration;
using Windows.Devices.HumanInterfaceDevice;
using Windows.Storage;
using Windows.UI.Xaml.Controls;
namespace HIDdeviceTest
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
EnumerateHidDevices();
}
// Find HID devices.
private async void EnumerateHidDevices()
{
// Microsoft Input Configuration Device.
ushort vendorId = 0x045E;
ushort productId = 0x07CD;
ushort usagePage = 0x000D;
ushort usageId = 0x000E;
// Create the selector.
string selector =
HidDevice.GetDeviceSelector(usagePage, usageId, vendorId, productId);
// Enumerate devices using the selector.
var devices = await DeviceInformation.FindAllAsync(selector);
if (devices.Any())
{
// At this point the device is available to communicate with
// So we can send/receive HID reports from it or
// query it for control descriptions.
info.Text = "HID devices found: " + devices.Count;
// Open the target HID device.
HidDevice device =
await HidDevice.FromIdAsync(devices.ElementAt(0).Id,
FileAccessMode.ReadWrite);
if (device != null)
{
// Input reports contain data from the device.
device.InputReportReceived += async (sender, args) =>
{
HidInputReport inputReport = args.Report;
IBuffer buffer = inputReport.Data;
// Create a DispatchedHandler as we are interracting with the UI directly and the
// thread that this function is running on might not be the UI thread;
// if a non-UI thread modifies the UI, an exception is thrown.
await this.Dispatcher.RunAsync(
CoreDispatcherPriority.Normal,
new DispatchedHandler(() =>
{
info.Text += "\nHID Input Report: " + inputReport.ToString() +
"\nTotal number of bytes received: " + buffer.Length.ToString();
}));
};
}
}
else
{
// There were no HID devices that met the selector criteria.
info.Text = "HID device not found";
}
}
}
}
注釈
制限事項など、このクラスの使用方法の詳細については、「 ヒューマン インターフェイス デバイス (HID) のサポート 」および 「カスタム HID デバイスのサンプル」を参照してください。
このクラスを使用して HID デバイスにアクセスするアプリは、マニフェストの Capabilities ノードに特定の DeviceCapability データを含める必要があります。 このデータは、デバイスとその目的 (または機能) を識別します。 詳細については、「HID の デバイス機能を指定する方法」を参照してください。
プロパティ
ProductId |
指定された HID デバイスの製品識別子を取得します。 |
UsageId |
指定された HID デバイスの使用状況識別子を取得します。 |
UsagePage |
最上位のコレクションの使用状況ページを取得します。 |
VendorId |
指定された HID デバイスのベンダー識別子を取得します。 |
Version |
指定された HID デバイスのバージョン (リビジョン) 番号を取得します。 |
メソッド
Close() |
ホストと指定された HID デバイスの間の接続を閉じます。 |
CreateFeatureReport() |
ホストがデバイスに送信する唯一の機能レポート (既定) を作成します。 |
CreateFeatureReport(UInt16) |
ホストがデバイスに送信する reportId パラメーターで識別される機能レポートを作成します。 |
CreateOutputReport() |
ホストがデバイスに送信する唯一の既定の出力レポートを作成します。 |
CreateOutputReport(UInt16) |
ホストがデバイスに送信する reportId パラメーターで識別される出力レポートを作成します。 |
Dispose() |
アンマネージ リソースの解放またはリセットに関連付けられているアプリケーション定義のタスクを実行します。 |
FromIdAsync(String, FileAccessMode) |
deviceId パラメーターで識別されるデバイスへのハンドルを開きます。 アクセスの種類は、 accessMode パラメーターによって指定されます。 |
GetBooleanControlDescriptions(HidReportType, UInt16, UInt16) |
指定された HID デバイスのブール値コントロールの説明を取得します。 |
GetDeviceSelector(UInt16, UInt16) |
指定された usagePage と usageId に基づいて、高度なクエリ構文 (AQS) 文字列を取得 します。 |
GetDeviceSelector(UInt16, UInt16, UInt16, UInt16) |
指定された usagePage、usageId、 vendorId、 productId に基づいて、高度なクエリ構文 (AQS) 文字列を取得 します。 |
GetFeatureReportAsync() |
特定の HID デバイスから最初の機能レポート (既定) を非同期的に取得します。 |
GetFeatureReportAsync(UInt16) |
指定された HID デバイスの reportId パラメーターで識別される機能レポートを非同期的に取得します。 |
GetInputReportAsync() |
指定された HID デバイスから既定の入力レポート (最初) を非同期的に取得します。 |
GetInputReportAsync(UInt16) |
指定された HID デバイスから、 reportId パラメーターで識別される入力レポートを非同期的に取得します。 |
GetNumericControlDescriptions(HidReportType, UInt16, UInt16) |
指定された HID デバイスの数値コントロールの説明を取得します。 |
SendFeatureReportAsync(HidFeatureReport) |
ホストからデバイスに機能レポートを非同期的に送信します。 |
SendOutputReportAsync(HidOutputReport) |
出力レポートをホストからデバイスに非同期的に送信します。 |
イベント
InputReportReceived |
GetInputReportAsync() または GetInputReportAsync(System.UInt16 reportId) が呼び出されたときにデバイスによって発行された入力レポートを処理するイベント リスナーを確立します。 |