HidDevice 类
定义
重要
一些信息与预发行产品相关,相应产品在发行之前可能会进行重大修改。 对于此处提供的信息,Microsoft 不作任何明示或暗示的担保。
表示顶级集合和相应的设备。
public ref class HidDevice sealed : IClosable
/// [Windows.Foundation.Metadata.ContractVersion(Windows.Foundation.UniversalApiContract, 65536)]
/// [Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.Agile)]
class HidDevice final : IClosable
[Windows.Foundation.Metadata.ContractVersion(typeof(Windows.Foundation.UniversalApiContract), 65536)]
[Windows.Foundation.Metadata.MarshalingBehavior(Windows.Foundation.Metadata.MarshalingType.Agile)]
public sealed class HidDevice : System.IDisposable
Public NotInheritable Class HidDevice
Implements IDisposable
- 继承
- 属性
- 实现
Windows 要求
设备系列 |
Windows 10 (在 10.0.10240.0 - for Xbox, see UWP features that aren't yet supported on Xbox 中引入)
|
API contract |
Windows.Foundation.UniversalApiContract (在 v1.0 中引入)
|
示例
有关演示如何使用此类的完整示例,请参阅 自定义 HID 设备示例。
以下示例演示了使用 XAML 和 C# 生成的 UWP 应用如何使用 GetDeviceSelector 方法为特定 HID 设备创建选择器 (,在这种情况下,Microsoft 输入配置设备) 然后使用 FromIdAsync 方法打开与该设备的连接。
注意
此代码片段尝试查找系统中可能不存在的 HID 设备。 若要在系统上成功测试代码,应使用有效值更新 vendorId、productId、usagePage、usageId。
- 打开设备管理器
- 展开 人机接口设备
- 在本示例中找到 HID 设备 (,我们选择了 Microsoft 输入配置设备)
- 右键单击设备并选择 “属性”
- 在“属性”中,选择“ 详细信息 ”选项卡
- 在“详细信息”选项卡上,从“属性”下拉列表中选择“硬件 ID”
- HID 详细信息显示在 “值 ”框中:
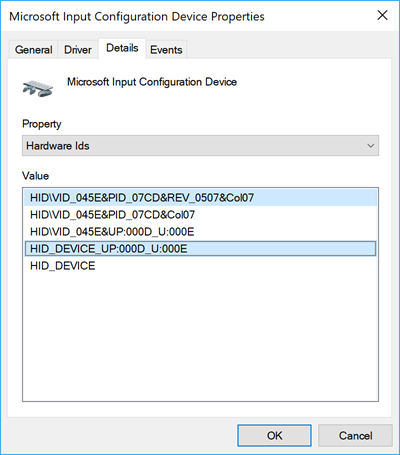
using System;
using System.Linq;
using Windows.Devices.Enumeration;
using Windows.Devices.HumanInterfaceDevice;
using Windows.Storage;
using Windows.UI.Xaml.Controls;
namespace HIDdeviceTest
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
EnumerateHidDevices();
}
// Find HID devices.
private async void EnumerateHidDevices()
{
// Microsoft Input Configuration Device.
ushort vendorId = 0x045E;
ushort productId = 0x07CD;
ushort usagePage = 0x000D;
ushort usageId = 0x000E;
// Create the selector.
string selector =
HidDevice.GetDeviceSelector(usagePage, usageId, vendorId, productId);
// Enumerate devices using the selector.
var devices = await DeviceInformation.FindAllAsync(selector);
if (devices.Any())
{
// At this point the device is available to communicate with
// So we can send/receive HID reports from it or
// query it for control descriptions.
info.Text = "HID devices found: " + devices.Count;
// Open the target HID device.
HidDevice device =
await HidDevice.FromIdAsync(devices.ElementAt(0).Id,
FileAccessMode.ReadWrite);
if (device != null)
{
// Input reports contain data from the device.
device.InputReportReceived += async (sender, args) =>
{
HidInputReport inputReport = args.Report;
IBuffer buffer = inputReport.Data;
// Create a DispatchedHandler as we are interracting with the UI directly and the
// thread that this function is running on might not be the UI thread;
// if a non-UI thread modifies the UI, an exception is thrown.
await this.Dispatcher.RunAsync(
CoreDispatcherPriority.Normal,
new DispatchedHandler(() =>
{
info.Text += "\nHID Input Report: " + inputReport.ToString() +
"\nTotal number of bytes received: " + buffer.Length.ToString();
}));
};
}
}
else
{
// There were no HID devices that met the selector criteria.
info.Text = "HID device not found";
}
}
}
}
注解
有关使用此类(包括限制)的详细信息,请参阅 支持人机接口设备 (HID) 和 自定义 HID 设备示例。
使用此类访问 HID 设备的应用必须在其清单的“功能”节点中包含特定的 DeviceCapability 数据。 此数据标识设备及其用途 (或功能) 。 有关详细信息,请参阅 如何为 HID 指定设备功能。
属性
ProductId |
获取给定 HID 设备的产品标识符。 |
UsageId |
获取给定 HID 设备的使用标识符。 |
UsagePage |
获取顶级集合的使用情况页。 |
VendorId |
获取给定 HID 设备的供应商标识符。 |
Version |
获取给定 HID 设备的版本或修订版本号。 |
方法
Close() |
关闭主机与给定 HID 设备之间的连接。 |
CreateFeatureReport() |
创建主机将发送到设备的唯一或默认功能报告。 |
CreateFeatureReport(UInt16) |
创建由 reportId 参数标识的功能报告,主机将发送到设备。 |
CreateOutputReport() |
创建主机将发送到设备的唯一或默认输出报告。 |
CreateOutputReport(UInt16) |
创建主机将发送到设备的输出报表(由 reportId 参数标识)。 |
Dispose() |
执行与释放或重置非托管资源关联的应用程序定义的任务。 |
FromIdAsync(String, FileAccessMode) |
打开由 deviceId 参数标识的设备句柄。 访问类型由 accessMode 参数指定。 |
GetBooleanControlDescriptions(HidReportType, UInt16, UInt16) |
检索给定 HID 设备的布尔控件的说明。 |
GetDeviceSelector(UInt16, UInt16) |
根据给定 usagePage 和 usageId 检索 AQS) 字符串 (高级查询语法。 |
GetDeviceSelector(UInt16, UInt16, UInt16, UInt16) |
根据给定 usagePage、 usageId、 vendorId 和 productId 检索 AQS) 字符串 (高级查询语法。 |
GetFeatureReportAsync() |
从给定 HID 设备异步检索第一个或默认功能报告。 |
GetFeatureReportAsync(UInt16) |
为给定 HID 设备异步检索 由 reportId 参数标识的特征报告。 |
GetInputReportAsync() |
从给定 HID 设备异步检索默认或第一个输入报告。 |
GetInputReportAsync(UInt16) |
从给定 HID 设备异步检索由 reportId 参数标识的输入报表。 |
GetNumericControlDescriptions(HidReportType, UInt16, UInt16) |
检索给定 HID 设备的数值控件的说明。 |
SendFeatureReportAsync(HidFeatureReport) |
以异步方式将功能报告从主机发送到设备。 |
SendOutputReportAsync(HidOutputReport) |
将输出报告从主机异步发送到设备。 |
事件
InputReportReceived |
建立一个事件侦听器,以处理在调用 System.UInt16 reportId) GetInputReportAsync () 或 GetInputReportAsync (时 设备发出的输入报告。 |